Design Pattern: What You Need to Know to Improve your Code Effectively!
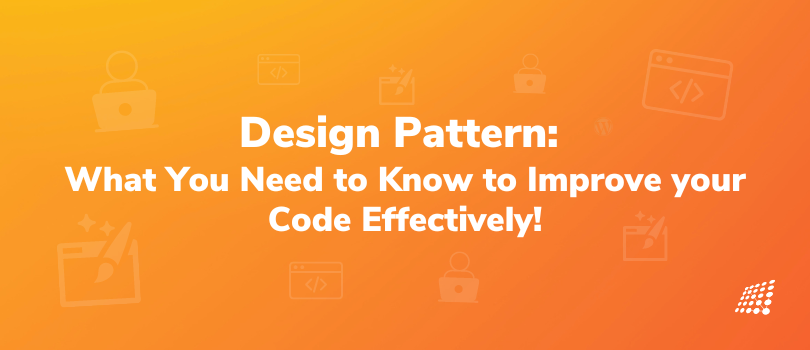
Are you tired of making the same errors in your software design? Do you want to improve your code and become a more efficient developer? Look no further than design patterns for efficient software development! You may be wondering, “what are design patterns in software development?” Well, these reusable solutions to common problems in software engineering act as a universal standard for solving issues that programmers face.
By using design patterns in software development, developers can communicate the structure of a software system to their team, allowing them to quickly and effectively build software with fewer mistakes. In this blog, we'll explore the upside of using design patterns, why they are essential to improving your code, the best design patterns for efficient code development, and some of the best design patterns for software development that every developer should know.
The Importance of Design Patterns in Software Engineering
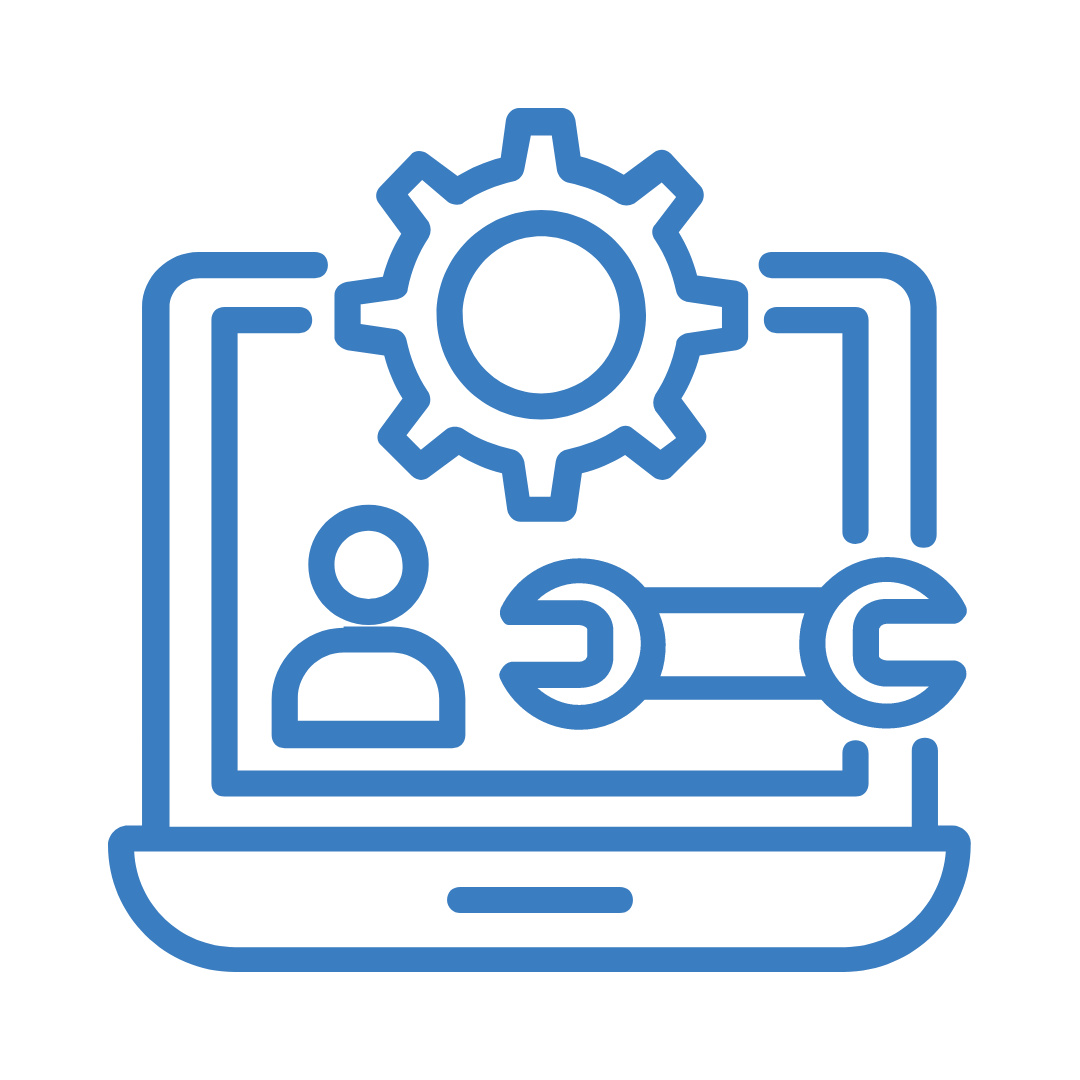
Now that you are aware of what are design patterns in software development, let’s move to why are design patterns important in software engineering?
- Design patterns are important because they provide a reliable, efficient, and reusable solution to common software development problems.
- They help developers focus on the problem at hand, rather than having to reinvent the wheel every time they encounter an issue.
- Design patterns also ensure that the code is more organized, maintainable,and easier to debug.
- Additionally, design patterns provide a language for developers to communicate their solutions to other developers so it becomes easier for developers to collaborate and build on each other's work.
These are just some of the benefits of design patterns in software development.
Some Design Patterns that Every Developer Should Know
Now, let’s explore some of the commonly used design patterns in software development.
1. Factory Design Pattern:
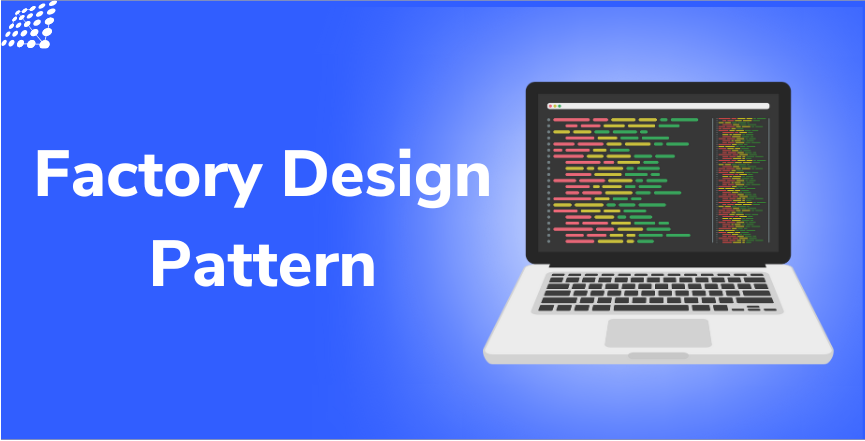
One of the essential design patterns for software development is the Factory Design Pattern which is a creational design pattern that encapsulates object creation. It involves a single class that is responsible for creating objects of other types. This class is called a factory”, and it contains methods that create objects of other types.
The Factory pattern is most useful when a system is decoupled into two parts: the main application and the object creators. That way, the main application can be unaware of the concrete classes used to create objects. The responsibility of creating objects is delegated to the factory class, making it easier to manage object creation and provide flexibility in object instantiation. Understanding factory design pattern is key to utilizing this powerful approach in your software development projects.
The Factory Design Pattern is used when the creation of objects becomes too complex for a single class to handle. If you're wondering "what is factory design pattern and how it works," the factory will handle the creation of objects by taking care of the details, such as choosing which type of object should be created and handling the construction of the object. So, instead of having to create an object directly, you can delegate that responsibility to a factory, which can simplify your code and make it more manageable.
Advantages of the Factory Design Pattern:
There are advantages of the factory design pattern you need to know about! The factory design pattern:
- Allows the system to be loosely coupled and easily extendable
- Hides the complexity of object creation from the client
- Enables the creation of objects without the need for the client to know the details of the object creation process
- Allows the system to be easily changed and maintained
- Allows for the reuse of existing objects
2. Builder Design Pattern:
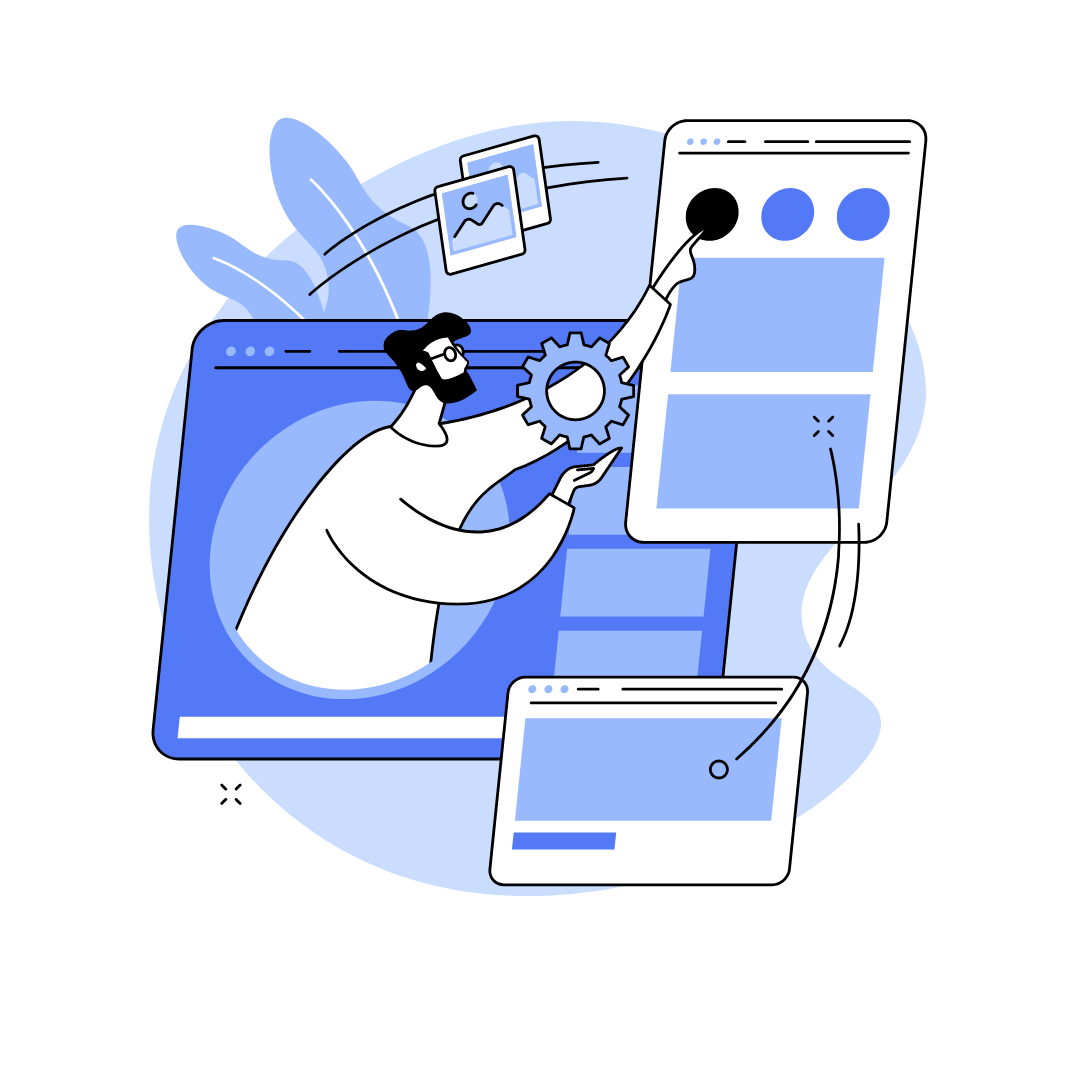
What is Builder design pattern and how does it work? The Builder design pattern is a creational design pattern that is used to create complex objects with simple interfaces, including Building Objects with Builder Design Pattern. It provides a step-by-step approach to constructing objects, allowing for the creation of complex objects without having to write complex code. The Builder pattern is also useful for when you want to create objects with varying configurations and properties.
The four parts of Builder Design Pattern
- The Builder: This is an interface that defines the methods needed to construct the object.
- The Concrete Builder: This is a concrete class that implements the Builder interface, providing the specific implementation needed to construct the object.
- The Director: This is a class that is responsible for constructing the object using the Builder interface.
- The Product: This is the object that is being constructed by the Builder. The Builder pattern is useful when you want to create objects with many different properties and configurations. It allows for a flexible and modular way to create objects with complex logic.
3. Observer Design Pattern:
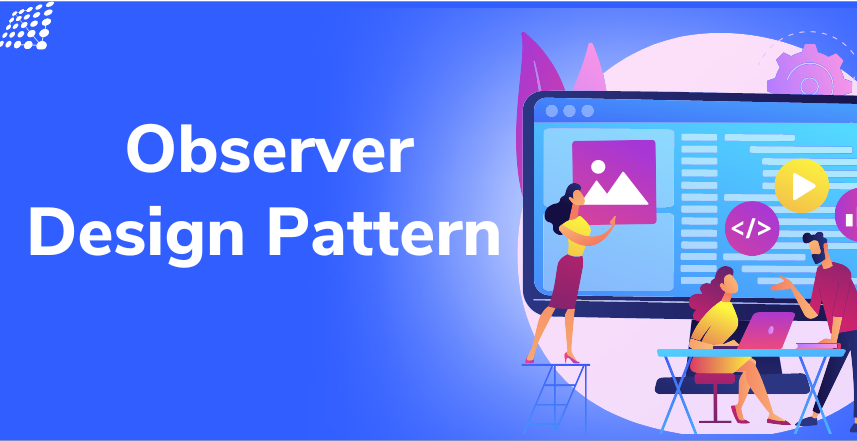
What is the Observer Design Pattern? A powerful behavioral design pattern that enables efficiently managing state changes with observer pattern. It enables efficient management of state changes, especially when dealing with a one-to-many relationship with observer design pattern.
It allows an object, known as the subject, to be observed by multiple observers, forming a one-to-many relationship. Whenever the state of the subject changes, all of its observers are notified and updated with the new information. This makes the Observer Pattern ideal for situations where multiple objects need to be aware of changes in another object, such as in user interfaces, where changes in data need to be reflected in multiple views. With the Observer Pattern, you can ensure that your code is organized, efficient, and easy to maintain, making it a valuable tool for any developer.
4. Iterator Design Pattern
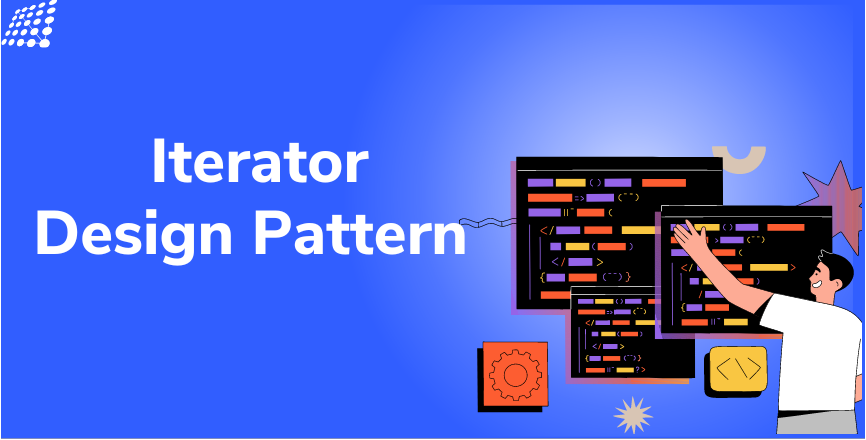
What is iterator design pattern and how it works? The Iterator Design Pattern is a behavioral design pattern that defines an interface for accessing and traversing elements of a collection without exposing its underlying representation. This pattern is particularly useful when working with collections of objects, as it provides a standard way to traverse through them without having to know their internal structure.
Using the Iterator Design Pattern with sequential access allows you to access each element in a collection sequentially, without having to explicitly manage the position of the iterator. Additionally, using the Iterator Pattern with sequential access in combination with the design pattern of sequential access with iterator, provides an even more efficient and effective way of accessing and manipulating the elements of a collection.
By implementing the Iterator Design Pattern with sequential access and the design pattern of sequential access with iterator, you can easily iterate through and manipulate any collection of objects with ease and without having to worry about their underlying structure.
The four elements of iterator design pattern:
- Iterator: This is an interface that defines methods for accessing and traversing elements of a collection.
- Concrete Iterator: This is a class that implements the Iterator interface and maintains the current position of the iterator.
- Aggregate: This is an interface that defines methods for creating and returning iterators.
- Concrete Aggregate: This is a class that implements the Aggregate interface and creates and returns iterators. The Iterator Pattern is often used in situations where you need to iterate over a collection of objects, but don't want to expose the internal structure of the collection. For example, you may have a collection of objects that represents a list of items, and you want to be able to iterate over the list.
5. Strategy Design Pattern:
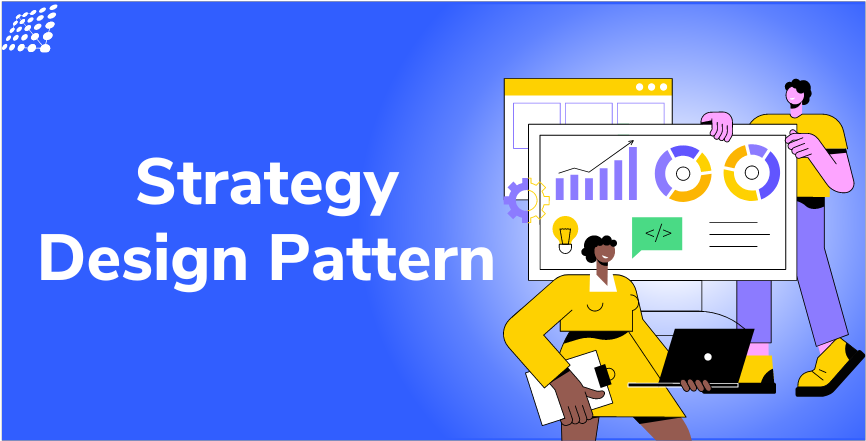
What is strategy design pattern and how it works? The Strategy Pattern, also known as the Policy Pattern, is a powerful tool in software engineering that can help you achieve the independence of algorithms with strategy design pattern. This pattern allows the algorithms to vary independently from the clients that use them.
The strategy pattern in software engineering is also known as the Policy Pattern. It is a behavioral design pattern that enables selecting an algorithm at runtime. Instead of implementing a single algorithm directly, code receives run-time instructions as to which algorithm in a family to use.
Strategy pattern is used when we have multiple algorithms for a specific task and the client decides the actual implementation to be used at runtime.
Example of Strategy pattern is given below:
An example of the Strategy pattern is an online shopping cart. The cart is the context, and the strategies are different payment methods such as credit card, PayPal, and cash on delivery. The client can select the payment method at checkout.
6. Adapter Design Pattern:
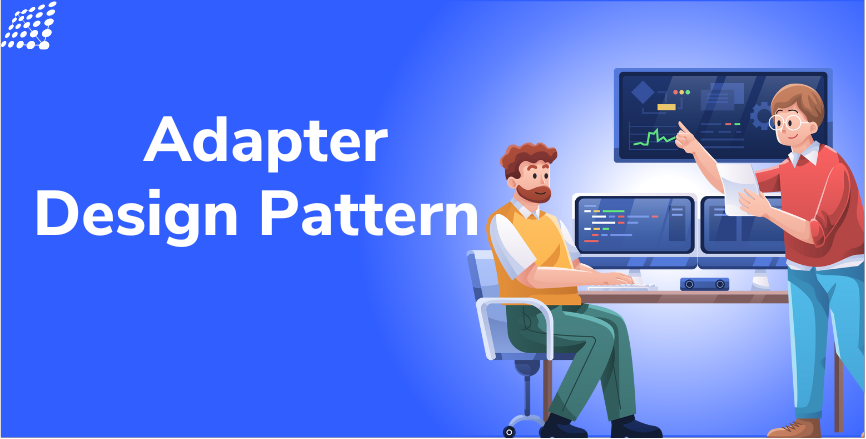
What is the adapter design pattern? The Adapter design pattern is a structural design pattern that is used to allow two incompatible objects to work together. It is also known as the Wrapper pattern and is used to convert the interface of one object to match that of another. This allows code written to work with one object to also work with another, even if the two objects are not compatible. The adapter acts as a bridge between the two incompatible objects, providing a common interface for both objects.
Now that you know what is adapter design pattern in software engineering, lets check out its participants.
The Adapter pattern has four participants:
• Target: This is the class that your client code expects.
• Adaptee: This is the class that needs adapting.
• Adapter: This is the adapter class that adapts the Adapter to the Target interface.
• Client: This is the client code that uses the Target interface.
There are benefits of using adapter pattern in software development. The adapter pattern is useful when you want to use an existing class, but it does not provide the interface that your client code expects. In this case, you can create an adapter class that adapts the existing class to the interface expected by your client code.
7. Facade design pattern:
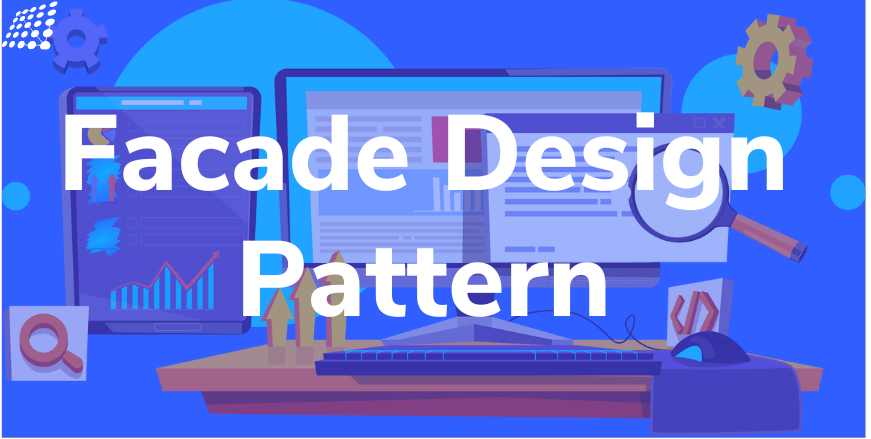
What is the facade pattern and why is it useful? The Facade Design Pattern is a structural design pattern that provides a simplified interface to a complex subsystem. It is used to hide the complexities of the subsystem from the client. The facade class provides a single interface to the complex logic of one or more classes. It is used to introduce an additional layer of abstraction between the client and the subsystem. The facade class can also provide additional functionality to the client, such as caching and performance optimization.
Finally, there are so many patterns you can find on Google that are used to solve a particular recurring problem in your codebase. So that you can make your codebase more reusable, and when many developers work on a project, the consistency of your codebase will be good for the long run. Also, any developer can easily find out the actual problem, so you can solve your client’s problem in a short amount of time as well.
Now all that is left for you to do is take your software engineering skills to the next level. To learn more on how to improve your code and get the best design patterns for efficient code development, get in touch with our web development company in New York.
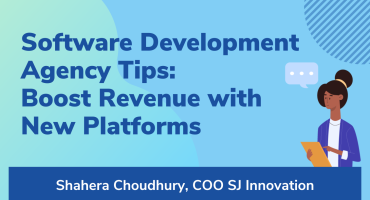
Software Development Agency Tips: Boost Revenue with New Platforms
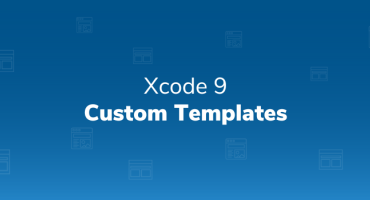
Xcode 9 Custom Templates
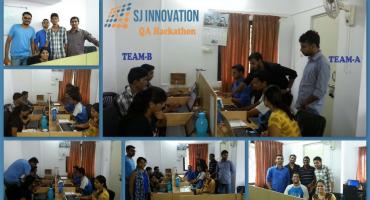